Inheritance
Inheritance allows a class to inherit properties and behaviors from another class. Inheritance is implemented through classes, creating a relationship between a base class (also known as a parent class) and a derived class (also known as a child class). The derived class inherits attributes and methods from the base class, promoting code reuse and extending functionality.
Base Class (Parent Class):
- The class whose properties and behaviors are inherited by another class is called the base class or parent class.
- It serves as a template for creating more specialized classes.
Derived Class (Child Class):
- The class that inherits from another class is called the derived class or child class.
- It extends or modifies the properties and behaviors inherited from the base class.
Access Modifiers:
- Public Inheritance (
public
):- When a derived class inherits publicly from a base class, the public members of the base class remain public in the derived class.
- Public inheritance establishes an "is-a" relationship, indicating that the derived class is a specialized form of the base class.
- Protected Inheritance (
protected
):- In the case of protected inheritance, the public members of the base class become protected in the derived class, and protected members remain protected.
- Protected inheritance is less common and is used when a derived class is expected to have a "has-a" or "implemented-in-terms-of" relationship with the base class.
- Private Inheritance (
private
):- With private inheritance, both public and protected members of the base class become private in the derived class.
- Private inheritance is used when the derived class needs to implement certain functionalities of the base class without exposing the interface of the base class to the outside world.
Types of Inheritance:
- Single Inheritance:
- In single inheritance, a derived class inherits from only one base class.
- It establishes a linear relationship between classes, and the derived class acquires the properties and behaviors of a single base class.
- Multiple Inheritance:
- Multiple inheritance allows a derived class to inherit from more than one base class.
- The derived class incorporates properties and behaviors from multiple base classes
- Multilevel Inheritance:
- Multilevel inheritance involves a chain of inheritance with multiple levels of derivation.
- A class is derived from another class, and then a new class is derived from the intermediate class.
- Hierarchical Inheritance:
- In hierarchical inheritance, multiple derived classes inherit from a single base class.
- Hybrid Inheritance:
- Hybrid inheritance is a combination of two or more types of inheritance.
- It may include a mix of single, multiple, multilevel, and hierarchical inheritance within the same class hierarchy.
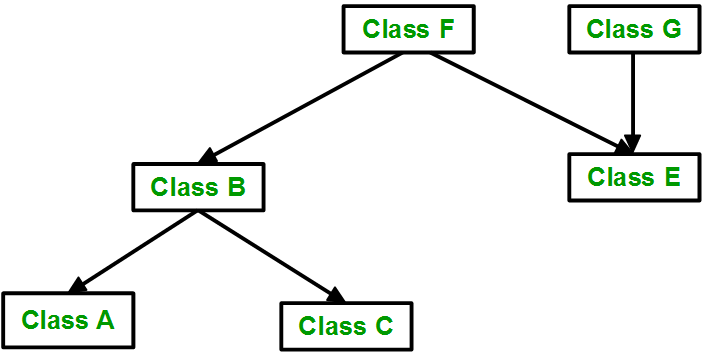
- Multipath Inheritance(Virtual Base class):
- In multipath inheritance, a derived class inherits from a common base class through multiple routes in the class hierarchy.
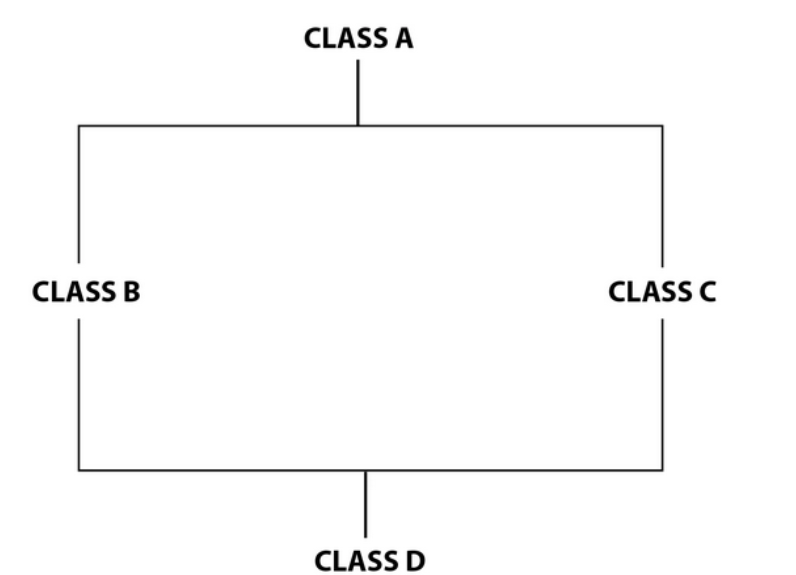
Ambiguity:
- The main issue with multipath inheritance is ambiguity, especially when there are common methods or attributes in the base class.
- When a derived class attempts to access a method or attribute from the common base class, there might be confusion about which path to follow.
Solutions:
- Virtual Inheritance: Use virtual inheritance to ensure that there is only one instance of the common base class shared among the derived classes. This resolves the ambiguity by creating a shared base class subobject.
- Explicit Path Specification: When calling methods, explicitly specify the path from the derived class to the desired base class to resolve ambiguity.